This article is basically my findings in building an SPA without a framework or library.
Building web applications as Single Page Applictions (SPAs) is a big trend today with the SPA mode being defacto for most frameworks. Frontend frameworks like React and Vue.js provide you with create-react-app
or create-vue-app
respectively to easily give a headstart with less time to setup.
The number of people on this bandwagon is huge and unfortunately a lack of understanding of the underlying magic
to these tools contribute to poor user experiences in product performance and accessibility of applications built with this approach.
In-depth understanding of how SPAs are coupled can yield better product experiences and a lot of this can be achieved by going in-depth into how configurations, templates, and module bundling work.
In every SPA, there is usually an entry point where every other dependency is added in their order of need. This controller sometimes acts as a router to different components needed, or in some cases it just starts the train of events of transitioning between the different components. But there is a little of problem what will manage the bundling of these components in their order?
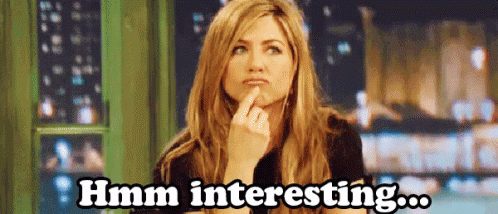
Welcome to the Webpack Show. Webpack is an open source JavaScript module bundler. Below is an image from the webpack website that explains how it works.
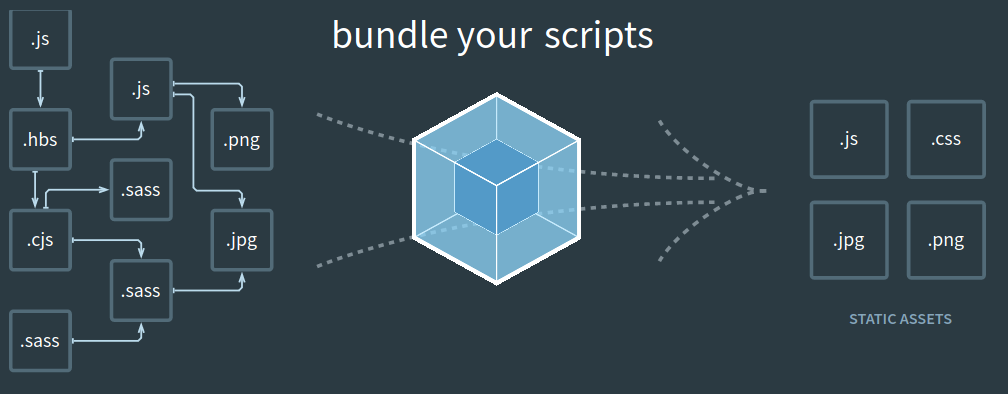
I get ideas of what is essential when packing my suitcase
Diane von Fürstenberg
From the image above it can be seen that webpack does not just bundle scripts. Since the app is controlled by javascript, webpack takes care of any imported module including images, stylesheets, etc. The remarkable thing about webpack is the quote above, webpack when configured with the proper plugins and loaders optimizes the codebase to the best; removing unused code, code splitting and compression of all other assets including CSS.
We have managed to solve our problem with webpack, now we can comfortably build a proper static app with all the optimization stated above. But it is obvious that most of the components we will work with will be dynamic components. This poses a new problem; while it might be possible to do this with the appending and prepending of HTML elements and playing with HTML strings, this approach would not make for clean code.
The most efficient way to handle a component approach in a clean way would be to utilise TEMPLATING
TEMPLATING is a document or file having a preset format, used as a starting point for a particular application so that the format does not have to be recreated each time it is used. There are many client side templating engines but for this project I used the nunjucks template engine because it more sophisticated than the rest of the competition.
Nunjucks templating is well documented and can easily be followed. For instance the example code for looping through contents can be found below
var items = [{ title: "foo", id: 1 }, { title: "bar", id: 2}];
<h1>Posts</h1>
<ul>
{% for item in items %}
<li>{{ item.title }}</li>
{% else %}
<li>This would display if the 'item' collection were empty</li>
{% endfor %}
</ul>
Webpack also has a loader for nunjucks files, it takes care of the importing of the template and prepares it for rendering. with this the app can easily be broken into components and easily imported when needed.
In summary, webpack and the use of templates for componentizing an app can summarized in an anonymous quote I came across recently
Cleaning out the past, Packing the present, and Preparing for a much Better future
In the second part of this article we'll utilize our in-depth understanding of webpack and nunjucks to build a small, componentized, and much more performant SPA than you'll get with a cookie-cutter approach typically recommend with off-the-shelf frameworks.